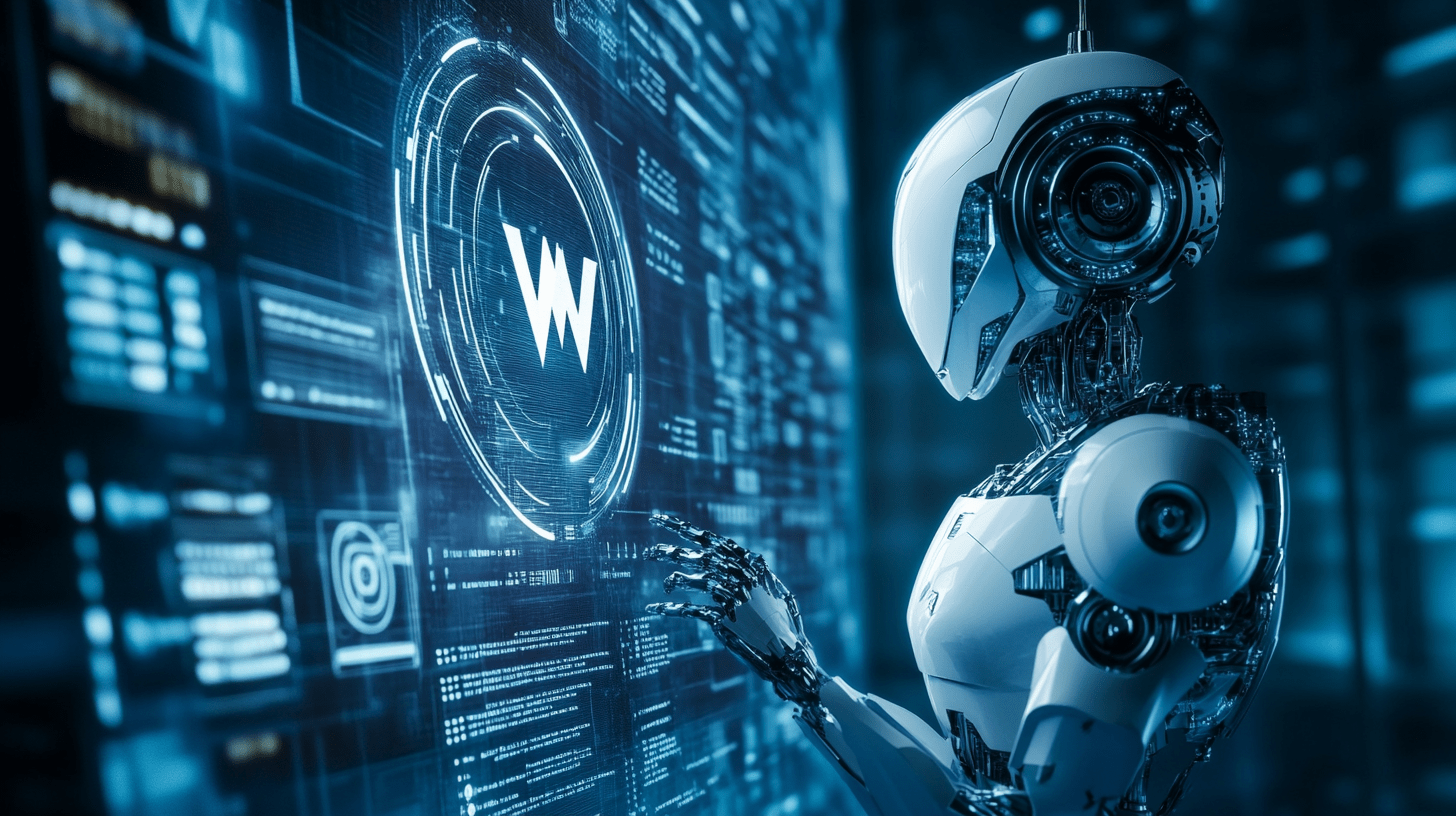
How to Use Next.js for High-Performance Web Applications
Learn the ins and outs of using Next.js to build fast, SEO-friendly web applications with Ben Bond's guidance.
How to Use Next.js for High-Performance Web Applications
In the fast-paced world of web development, performance is king. Whether you're a developer or a business owner looking to upgrade your site's capabilities, understanding how to harness the power of Next.js can be a game-changer. Next.js, a React framework developed by Vercel, is designed to give you the best of both worlds: the flexibility of React with the performance benefits of server-side rendering. In this comprehensive guide, I, Ben Bond, will walk you through how to use Next.js to build high-performance web applications that not only load quickly but also provide a seamless user experience.
Why Next.js for High-Performance Web Apps?
Before diving into the technicalities, let's explore why Next.js has become a go-to choice for developers:
Server-Side Rendering (SSR): Next.js allows you to pre-render pages on the server, which can significantly improve the initial load time of your web pages. This is particularly beneficial for SEO, as search engines can crawl the site more effectively.
Static Generation: With Next.js, you can generate static HTML at build time, which can be served directly by CDN, reducing server load and increasing speed.
Automatic Code Splitting: Next.js automatically splits your JavaScript bundle into smaller chunks, loading only what's necessary for each page.
Route Prefetching: Next.js can prefetch pages in the background, making navigation between pages feel instant.
Development Experience: Features like hot module replacement, zero configuration, and built-in CSS support make development smoother and faster.
How My Team and I Use Next.js
At Ben Bond Development, we leverage Next.js in various projects, from small business websites to complex web applications. Here’s how:
1. SEO Optimization: We ensure that your Next.js application is optimized for SEO from the ground up. This includes:
- Static Site Generation for pages that don't require dynamic content, which helps in reducing server load and improving SEO.
- Dynamic Routes for content that changes frequently, allowing for real-time updates while maintaining performance.
Learn more about SEO optimization in Next.js.
2. Performance Tuning:
- Lazy Loading: We implement lazy loading for images and other resources to ensure that only necessary assets are loaded initially.
- Caching Strategies: Utilizing Next.js's caching capabilities to reduce the load on your server, thereby speeding up page loads.
3. Integration with AI:
- AI-driven Content Generation: We use AI tools to automate content creation, reducing development time and ensuring content is always fresh and SEO-friendly. Explore AI tools for WordPress development.
- Personalization: Next.js allows for dynamic content loading, which we can use to deliver personalized user experiences with AI.
See how we integrate AI into Next.js applications.
Getting Started with Next.js
Setting Up Your Development Environment
To start with Next.js, you'll need:
- Node.js (preferably the latest LTS version)
- A code editor like Visual Studio Code
Here's a quick rundown on how to set up:
npx create-next-app@latest my-next-app
cd my-next-app
npm run dev
This command will create a new Next.js project. Now, let's dive deeper:
Create Pages: Each file in the
pages
directory becomes a route in your application. For instance,pages/about.js
will be accessible at/about
.Dynamic Routes: Use square brackets for dynamic routes, e.g.,
pages/post/[id].js
to handle individual blog posts.Custom App and Document: Modify
_app.js
and_document.js
for global configurations.
Key Features for Performance
Server-Side Rendering
export async function getServerSideProps(context) {
// Fetch data from external API
const res = await fetch(`https://.../data`)
const data = await res.json()
// Pass data to the page via props
return { props: { data } }
}
This function runs on the server and can fetch data at request time, ensuring your pages are always up to date.
Static Generation
export async function getStaticProps() {
const res = await fetch('https://.../posts')
const posts = await res.json()
return {
props: {
posts,
},
revalidate: 10, // In seconds
}
}
Here, pages are generated at build time but can be revalidated periodically for fresh content.
API Routes
Next.js allows you to create API endpoints directly in your project:
export default function handler(req, res) {
res.status(200).json({ text: 'Hello' });
}
This can be particularly useful for handling form submissions or server-side logic.
Advanced Next.js Techniques
- Middleware: Use middleware to run code before a request is completed, which can be useful for authentication or redirects.
- Incremental Static Regeneration: Rebuild parts of your site in the background, allowing for updates without full re-deployment.
Performance Optimization Tips
Here are some tips to ensure your Next.js application performs at its best:
- Minimize bundle size: Use tree shaking and only import what you need.
- Optimize images: Use Next.js's built-in
Image
component for automatic image optimization. - Leverage CDN: Serve your static files from a Content Delivery Network for faster content delivery.
- Code Splitting: Ensure that your code is split in a way that only loads what's necessary for each page.
Real-World Applications of Next.js
Case Studies
E-commerce Platform: We developed an e-commerce site where product pages are statically generated, but the inventory and pricing are updated dynamically. This approach reduces load times while ensuring real-time data accuracy.
Blogging Platform: For a client needing a fast, SEO-friendly blog, we used Next.js with dynamic routes to serve individual posts, integrating AI for content suggestions and personalization.
Learn how to build a high-performance e-commerce site.
Integrating with WordPress
Next.js can be used as a frontend for WordPress, creating a headless CMS setup:
- API Integration: Use WordPress's REST API to fetch content dynamically or generate static pages.
- SEO Benefits: Keep the SEO advantages of WordPress while enjoying Next.js's performance benefits.
Explore setting up a headless WordPress site with Next.js.
Conclusion
Next.js offers a powerful set of tools for developers to create high-performance web applications. From server-side rendering to static site generation, the framework provides the flexibility and speed needed for modern web development. My team and I at Ben Bond Development are here to help you leverage these capabilities to build fast, SEO-optimized, and user-friendly applications.
If you're considering upgrading your web application or starting a new project, let's discuss how Next.js can benefit your business. Visit our services page to learn more about what we offer, or get a quote for your project. For any questions or to start your journey with Next.js, feel free to contact us.
Remember, in the world of web development, performance is not just a feature; it's a necessity, and with Next.js, you're equipped to deliver just that.