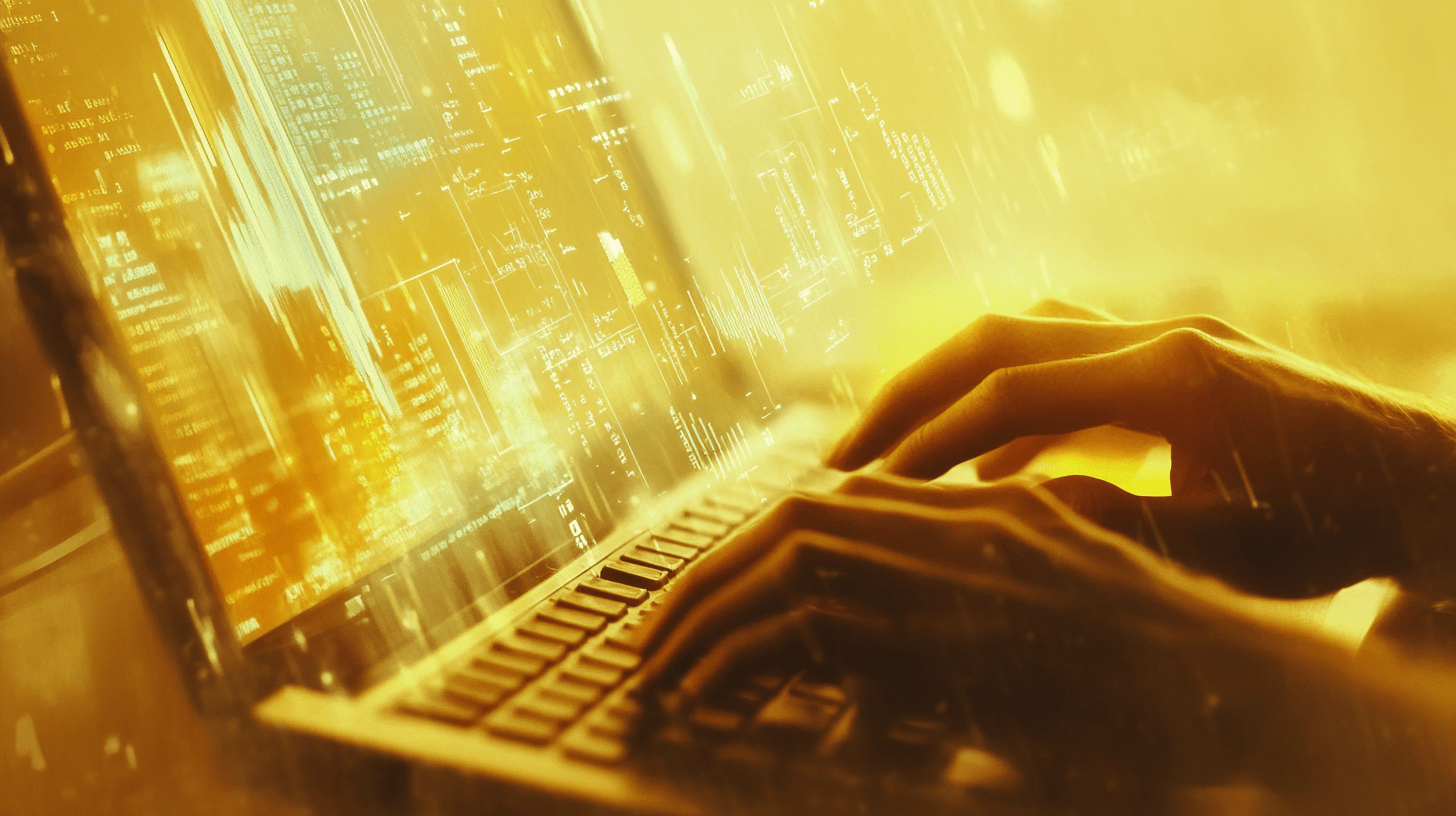
Best Practices for SEO Optimization in Next.js
In this comprehensive guide, Ben Bond shares expert insights on leveraging Next.js for SEO, covering everything from server-side rendering to AI integration.
Best Practices for SEO Optimization in Next.js
As a developer or business owner looking to enhance your web presence, understanding SEO optimization for Next.js can be a game changer. In this detailed guide, we'll explore how Next.js can be utilized to improve your site's SEO, ensuring you get the most out of your development efforts. My team and I at benbond.dev have extensive experience in this area, and I'm here to share some of the best practices that we've found to be effective.
Why Next.js for SEO?
Next.js, developed by Vercel, is a React framework that offers server-side rendering (SSR) and static site generation (SSG). Here's why it's a strong choice for SEO:
- Server-Side Rendering (SSR): Next.js can render pages on the server, which means search engines can crawl and index your content more efficiently.
- Static Site Generation (SSG): With SSG, you can generate static HTML files at build time, providing excellent performance and SEO benefits.
- Automatic Code Splitting: This feature ensures that only the necessary JavaScript is sent to the client, reducing load times and improving SEO scores.
Key SEO Best Practices in Next.js
1. Implement Server-Side Rendering
Server-side rendering is crucial for SEO because it allows search engines to see the fully rendered page. Here’s how you can implement it:
export async function getServerSideProps(context) {
const data = await fetchData(); // Fetch your data here
return {
props: {
data
}
}
}
2. Use Static Site Generation for Static Content
For pages with static content that don't change frequently, SSG can be your best friend:
export async function getStaticProps() {
const data = await fetchStaticData();
return {
props: {
data
},
revalidate: 10 // In seconds, how often to revalidate the page
}
}
3. Optimize for Speed
Speed is a key factor in SEO:
- Minimize JavaScript: Use Next.js's automatic code splitting.
- Optimize Images: Implement lazy loading for images (
loading="lazy"
). - Use Next.js Image Component: This component optimizes images automatically:
import Image from 'next/image'
function HomePage() {
return <Image src="/example.jpg" alt="Example" width={300} height={200} />
}
4. Properly Handle URL Structures
- Dynamic Routes: Use dynamic routes to create SEO-friendly URLs.
// pages/blog/[slug].js
import { useRouter } from 'next/router'
export default function BlogPost() {
const router = useRouter()
const { slug } = router.query
return <h1>{slug}</h1>
}
- Canonical URLs: If you have duplicate content, specify canonical URLs to avoid SEO penalties.
5. Leverage Next.js SEO Components
Next.js provides components like Head
for SEO:
import Head from 'next/head'
export default function Home() {
return (
<div>
<Head>
<title>My Page Title</title>
<meta name="description" content="A brief description of my page" />
</Head>
<h1>Welcome to My Next.js Site</h1>
{/* ... */}
</div>
)
}
6. Implement Schema Markup
Schema markup helps search engines understand your content better:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "Article Headline",
"image": ["URL of the image"],
"author": "Author's name",
"publisher": {
"@type": "Organization",
"name": "Your Company Name",
"logo": {
"@type": "ImageObject",
"url": "URL of your logo"
}
}
}
</script>
7. Use AI for Content Optimization
Integrating AI can significantly boost your SEO efforts:
- Content Generation: AI can help generate content that aligns with SEO best practices. Check out our guide on [/blog/using-openai-for-advanced-content-generation-in-wordpress] for more insights.
- Keyword Analysis: AI tools can analyze search trends and optimize keywords dynamically.
8. Structured Data for Enhanced Search Results
Implement structured data to enhance your search results:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "WebSite",
"url": "https://benbond.dev/",
"name": "Ben Bond's Web Development Services",
"description": "Expert WordPress, Next.js, and AI-driven solutions for your web needs."
}
</script>
9. Monitor and Analyze with AI
Use AI to:
- Track SEO Performance: AI can analyze your site's performance, providing insights on SEO health. Learn more about this in our article on [/blog/using-ai-to-track-content-performance-in-wordpress].
- A/B Testing: Implement AI-driven A/B testing to find the best SEO strategies. Explore our guide on [/blog/using-ai-for-improved-a-b-testing-in-wordpress].
10. Content Freshness
Search engines favor fresh content:
- Regular Updates: Keep your content up to date.
- AI-driven Content Suggestions: Use AI to suggest updates or new content based on trends. Dive deeper into this topic with [/blog/using-ai-for-dynamic-content-suggestions-in-wordpress].
Conclusion
Optimizing SEO in Next.js isn't just about following a checklist; it's about understanding how Next.js can work in harmony with SEO principles to deliver a site that's not only fast and user-friendly but also highly visible to search engines. My team and I at benbond.dev are committed to providing you with top-notch services to help you achieve this. Whether you're looking for a quote or need to contact us for tailored solutions, we're here to help you leverage the full potential of Next.js for your SEO needs.
By applying these best practices, you're setting up your Next.js site for SEO success, ensuring it ranks well and attracts organic traffic. Remember, SEO is an ongoing process, and with the right tools and expertise, like what we offer at benbond.dev, you can keep your site at the forefront of search results.
Keep learning, keep optimizing, and let's build something great together!