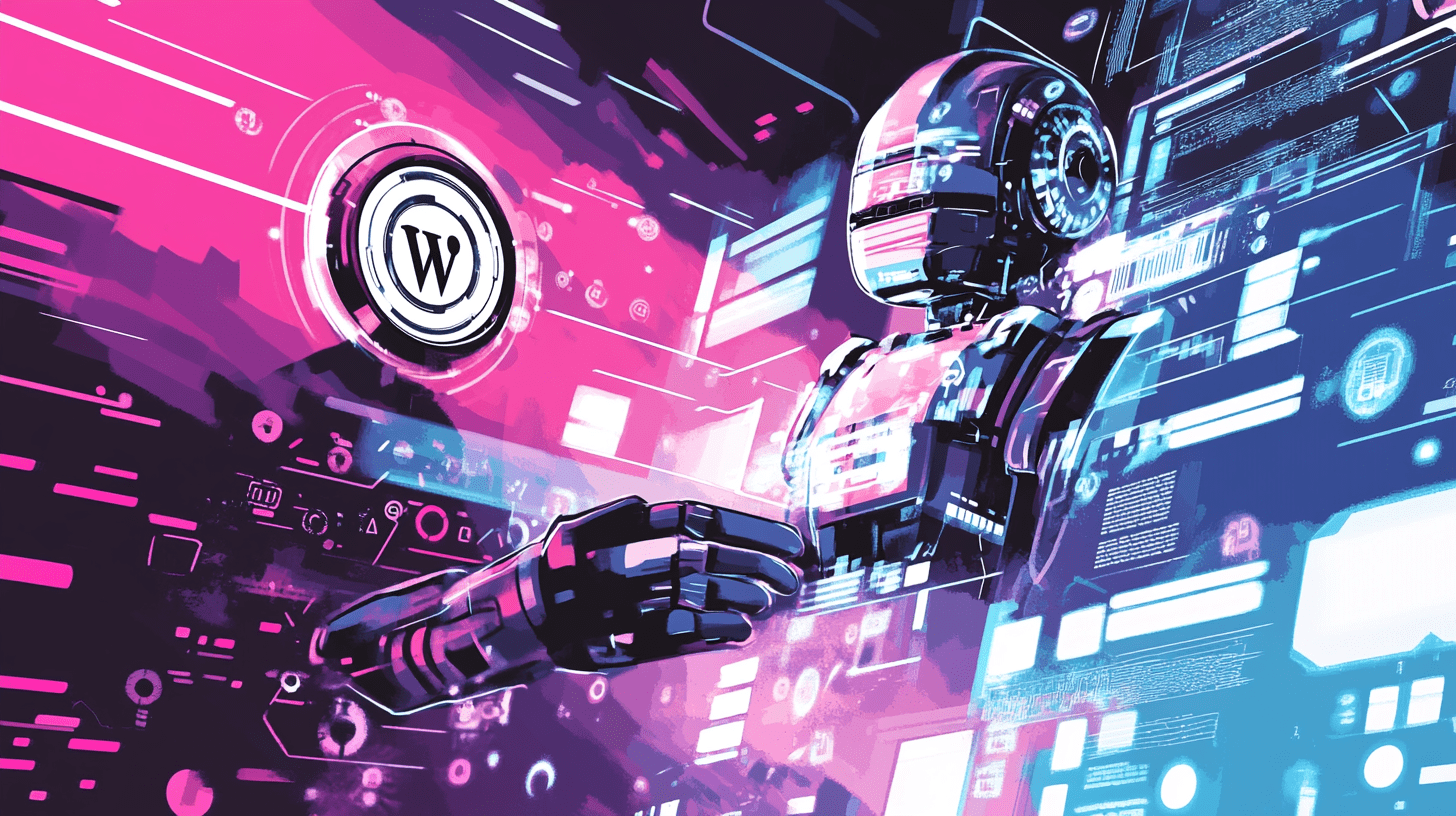
How to Build Scalable Web Applications with Next.js and AI
In this guide, Ben Bond explores the integration of Next.js with AI to create dynamic, scalable web applications, offering insights into best practices and real-world examples.
How to Build Scalable Web Applications with Next.js and AI
In today's fast-paced digital landscape, scalability isn't just a buzzword; it's a necessity. As businesses grow, so do their online platforms, and the need for a web application that can handle increased traffic, data, and complexity becomes paramount. That's where Next.js and AI come into play. In this comprehensive guide, we'll explore how to build scalable web applications using Next.js, enhanced by AI technologies, ensuring that your site can grow with your business.
Understanding Scalability in Web Development
Scalability refers to a system's ability to handle growth in terms of users, data, and functionality without degrading performance. Here are some key aspects:
- Vertical Scalability: Increasing the power of existing hardware resources.
- Horizontal Scalability: Adding more instances or nodes to distribute load.
- Database Scalability: Ensuring your database can manage increased data efficiently.
Why Next.js for Scalability?
Next.js, a React framework, offers several features that make it ideal for scalable web applications:
- Server-Side Rendering (SSR): Improves SEO and initial load time, crucial for handling traffic spikes.
- Static Site Generation (SSG): Pre-rendering pages at build time for faster load times.
- API Routes: Allows for serverless function development, reducing server load.
- Automatic Code Splitting: Loads only the necessary JavaScript, enhancing performance.
Integrating AI for Enhanced Scalability
AI isn't just about futuristic technology; it's about making your application smarter, more efficient, and user-friendly. Here's how AI can enhance scalability:
1. Content Generation and Optimization
Automated Content Creation: Use AI to generate content or ideas for content, reducing the need for manual input. Check out our guide on [/blog/using-ai-to-boost-wordpress-content-creation] to see how AI can help in this area.
SEO Optimization: AI can analyze trends, user behavior, and SEO metrics to optimize content automatically. Learn more about AI-powered SEO strategies in our article on [/blog/ai-powered-seo-strategies-for-next-js-sites].
2. User Experience Personalization
Personalized Recommendations: AI-driven systems can analyze user behavior to offer personalized content or product recommendations, enhancing user retention. Dive into how to implement this with our guide on [/blog/building-an-ai-powered-recommendation-engine-for-wordpress].
Dynamic Content: AI can dynamically adjust content based on user interactions, ensuring a tailored experience. Understand how to use AI for this in [/blog/using-ai-to-personalize-landing-pages-in-wordpress].
3. Performance Optimization
Load Balancing: AI algorithms can predict traffic patterns and manage load distribution effectively.
Caching Strategies: AI can help in deciding what to cache and for how long, optimizing server load. For more on caching, see [/blog/improving-wordpress-performance-with-next-js-server-side-rendering].
4. Security and Maintenance
Intrusion Detection: AI can monitor for unusual patterns that might indicate security breaches, protecting your application.
Automated Updates and Maintenance: AI can automate routine maintenance tasks, ensuring your application remains secure and up-to-date. For insights on this, visit [/blog/automating-wordpress-development-tasks-with-ai].
Building with Next.js and AI: A Practical Approach
Let's walk through a step-by-step approach to building a scalable web application:
Step 1: Project Setup
Start by setting up your Next.js project:
npx create-next-app my-scalable-app
cd my-scalable-app
Step 2: Integrate AI Services
Choose AI services that align with your application's needs:
- Natural Language Processing (NLP): For content generation or chatbots.
- Machine Learning: For predictive analytics or user behavior analysis.
Table: AI Services Comparison
Service | Use Case | Integration with Next.js |
---|---|---|
Google Cloud NLP | Content Analysis | API Routes |
AWS Comprehend | Sentiment Analysis | Serverless Functions |
Azure AI | Image Recognition | Edge Functions |
Hugging Face | Custom Models | Client-side Fetching |
Step 3: Develop Scalable Components
- Server-Side Rendering: Use SSR for pages that require real-time data or personalization:
export async function getServerSideProps(context) {
const data = await fetchUserData(context.query.userId);
return {
props: { data },
};
}
- Static Generation: For static content, use SSG to pre-render pages:
export async function getStaticProps() {
const posts = await fetchBlogPosts();
return {
props: { posts },
revalidate: 10, // Revalidate at most once every 10 seconds
};
}
Step 4: Optimize for Performance
- Code Splitting: Next.js automatically splits your code into smaller chunks, but you can further optimize:
// In your page component
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/HeavyComponent'), {
ssr: false,
});
- Lazy Loading: Implement lazy loading for images and other media to reduce initial load times:
import Image from 'next/image';
function LazyImage() {
return (
<Image
src="/path/to/image.jpg"
alt="Description"
width={500}
height={500}
loading="lazy"
/>
);
}
Step 5: Implement AI-Driven Features
- AI Chatbot: Integrate an AI-powered chatbot for customer support:
import { useState } from 'react';
function Chatbot() {
const [messages, setMessages] = useState([]);
const handleSendMessage = async (message) => {
const response = await fetch('/api/chatbot', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ message }),
});
const data = await response.json();
setMessages([...messages, { text: message, type: 'user' }, { text: data.response, type: 'ai' }]);
};
return (
<div>
{messages.map((msg, index) => (
<div key={index} className={msg.type === 'user' ? 'user-message' : 'ai-message'}>
{msg.text}
</div>
))}
<input type="text" onKeyPress={(e) => e.key === 'Enter' && handleSendMessage(e.target.value)} />
</div>
);
}
- Personalization: Use AI to tailor user experiences:
// In your API route
import { analyzeUserBehavior } from '../lib/ai';
export default async function handler(req, res) {
const userBehavior = await analyzeUserBehavior(req.body.userId);
const personalizedContent = generateContentBasedOnBehavior(userBehavior);
res.status(200).json(personalizedContent);
}
Step 6: Monitor and Scale
Use Monitoring Tools: Implement tools like New Relic or Datadog to keep an eye on your application's performance metrics.
Horizontal Scaling: Set up your application to scale out:
# Example with Kubernetes
kubectl scale deployment/my-app --replicas=5
Step 7: Continuous Integration and Deployment (CI/CD)
- Automate Testing: Use tools like Jest or Cypress to ensure your code works as expected:
npm install --save-dev jest cypress
- CI/CD Pipelines: Set up CI/CD pipelines with services like GitHub Actions or GitLab CI:
name: Deploy Next.js App
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
- run: npm ci
- run: npm run build
- run: npm run start
Conclusion
Building scalable web applications with Next.js and AI isn't just about leveraging technology; it's about creating an ecosystem where your application can grow seamlessly with your business. From content generation to performance optimization, AI enhances every aspect of your application, making it more dynamic and user-centric.
If you're looking to take your web development to the next level, my team and I at benbond.dev are here to help. With our expertise in WordPress, Next.js, and AI, we can guide you through the complexities of building scalable applications. Check out our services to see how we can assist you in scaling your online presence or request a quote for your project. For any questions or to discuss your project, feel free to contact us.
Remember, scalability is not a one-time effort but an ongoing journey. By integrating Next.js with AI, you're setting the foundation for a web application that can evolve with your needs, ensuring your digital platform remains robust, efficient, and user-friendly for years to come.