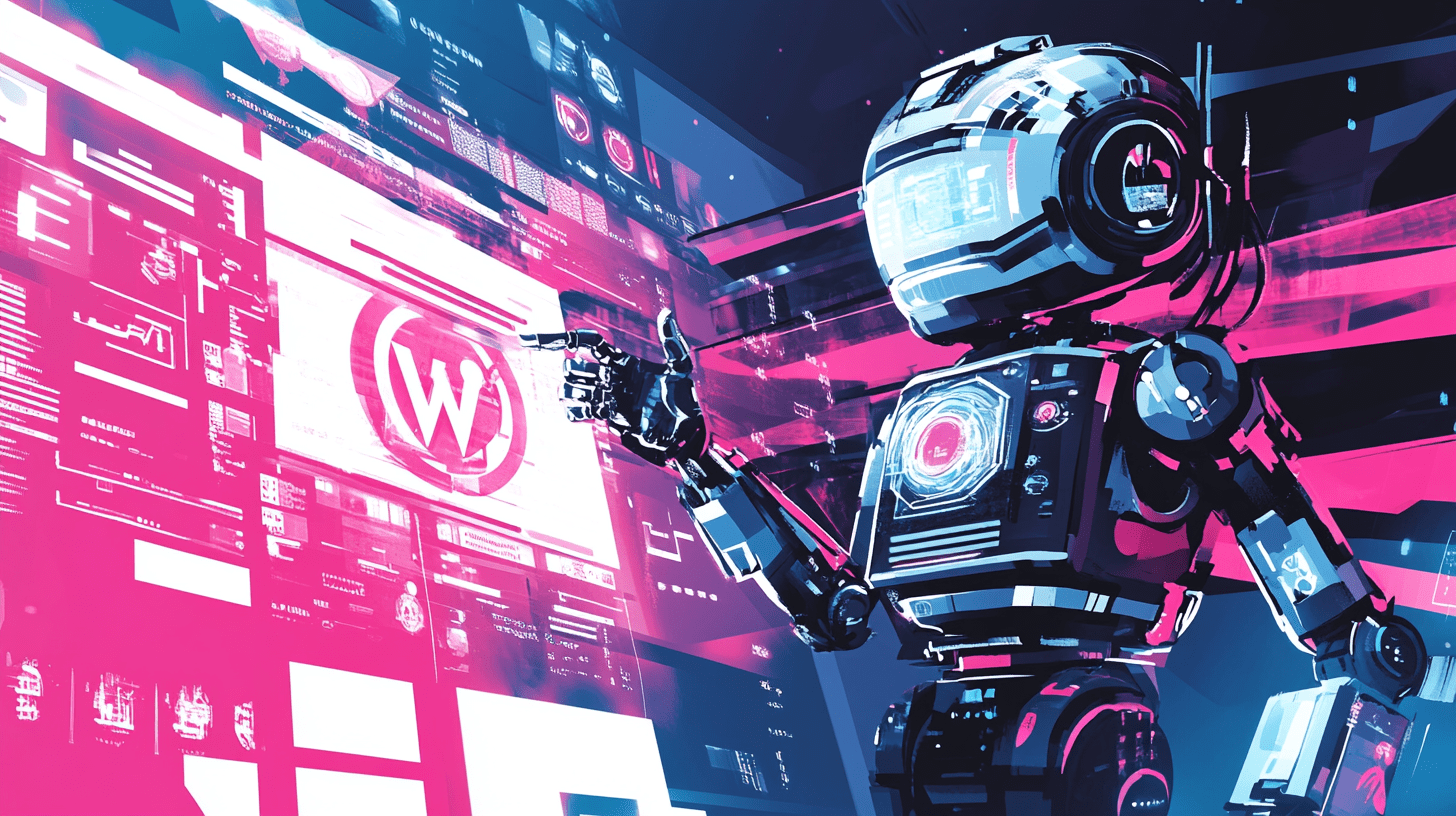
A Step-by-Step Guide to Building a Headless WordPress Site with Next.js
In this article, Ben Bond walks you through setting up a headless WordPress site using Next.js, offering insights into the benefits, and practical steps for implementation.
Introduction
Hey there, developers and business owners! Are you ready to dive into the world of modern web development? I'm Ben Bond, and today, I'm going to walk you through A Step-by-Step Guide to Building a Headless WordPress Site with Next.js. This isn't just another tutorial; it's your gateway to creating dynamic, fast, and scalable websites that leverage the power of WordPress's content management capabilities with the performance and flexibility of Next.js. Let's get started!
Why Go Headless with WordPress and Next.js?
Before we jump into the technicalities, let's understand why this combination is so powerful:
Flexibility: Decoupling the frontend from the backend allows for greater design freedom and the use of modern JavaScript frameworks like Next.js.
Performance: Next.js offers server-side rendering (SSR) and static site generation (SSG), which can significantly boost your site's speed and SEO performance.
Scalability: With a headless setup, you can scale your infrastructure independently of your content management system.
Enhanced User Experience: Next.js provides dynamic routing, optimized images, and better SEO out-of-the-box.
For more insights on why this is a game-changer, check out my post on how to build scalable web applications with Next.js and AI.
Setting Up Your WordPress Backend
Step 1: Install WordPress
If you haven't already, you'll need to install WordPress. Here's how:
Choose a Hosting Provider: Select a hosting service that supports PHP and MySQL. I recommend hosts like WP Engine or SiteGround for their WordPress-specific optimizations.
Download WordPress: Head over to wordpress.org and download the latest version.
Setup: Follow the installation instructions provided by your host or the WordPress Codex. Here's a quick rundown:
- Upload WordPress files to your server.
- Create a database for WordPress.
- Run the installation script by navigating to your domain.
Step 2: Configure WordPress for API Use
To use WordPress as a headless CMS:
Install WP REST API: This should be included by default in WordPress 4.7+, but ensure it's active.
Create Custom Post Types: If you need specialized content structures, define them using plugins or custom code. Here's a simple example:
function custom_post_type() {
register_post_type('books',
array(
'labels' => array(
'name' => __('Books'),
'singular_name' => __('Book')
),
'public' => true,
'has_archive' => true,
'show_in_rest' => true, // This line is crucial for REST API access
)
);
}
add_action('init', 'custom_post_type');
- Add Necessary Plugins: Consider plugins like WPGraphQL if you prefer GraphQL over REST API for better performance and flexibility.
Setting Up Next.js Frontend
Step 3: Create a Next.js Project
Install Node.js and npm: Ensure you have Node.js installed. You can download it from nodejs.org.
Initialize Next.js:
npx create-next-app@latest my-headless-site cd my-headless-site
Step 4: Integrate WordPress API
Fetch Data from WordPress: Use
getStaticProps
orgetServerSideProps
to fetch data from your WordPress REST API. Here's how you can do it:export async function getStaticProps() { const res = await fetch('YOUR_WORDPRESS_URL/wp-json/wp/v2/posts'); const posts = await res.json(); return { props: { posts, }, }; }
Display Data: Use the fetched data to dynamically generate pages. Here's a basic example for displaying posts:
import Head from 'next/head'; export default function Home({ posts }) { return ( <div className="container"> <Head> <title>My Headless WordPress Site</title> <link rel="icon" href="/favicon.ico" /> </Head> <main> <h1>Latest Posts</h1> <ul> {posts.map(post => ( <li key={post.id}>{post.title.rendered}</li> ))} </ul> </main> </div> ); }
Best Practices for Development
Use Environment Variables: Keep your WordPress URL and API keys secure with environment variables.
Leverage Next.js Features: Utilize Next.js's dynamic routing, API routes, and built-in optimizations like image optimization.
SEO Optimization: Ensure your site is SEO-friendly by using best practices for SEO optimization in Next.js.
Testing: Always test your site's performance, especially after fetching data from WordPress. Tools like Lighthouse can help.
Advanced Integration with AI
Now, let's talk about how AI can elevate your headless WordPress site:
Content Personalization: Use AI to dynamically adjust content based on user behavior. Check out AI-powered personalization in WordPress.
SEO Automation: Implement AI-driven SEO strategies to improve your site's visibility. Dive into AI-powered SEO strategies for Next.js sites.
User Experience: Enhance user interaction with AI-driven features like chatbots. Learn more at integrating AI-powered chatbots on your WordPress site.
Conclusion
Building a headless WordPress site with Next.js opens up a world of possibilities for developers and businesses looking to create high-performance, scalable websites. By following this guide, you've taken the first steps toward leveraging the best of both worlds: WordPress's robust content management with Next.js's modern web development capabilities.
If you're looking to take your web development to the next level, or need help with your project, my team and I are here to assist. Whether it's custom development services, a quick quote, or just some expert advice, feel free to contact us. Let's build something incredible together!
Remember, the future of web development is not just about coding; it's about creating experiences that resonate with users. With AI, WordPress, and Next.js, you're not just building a site; you're crafting a digital experience.