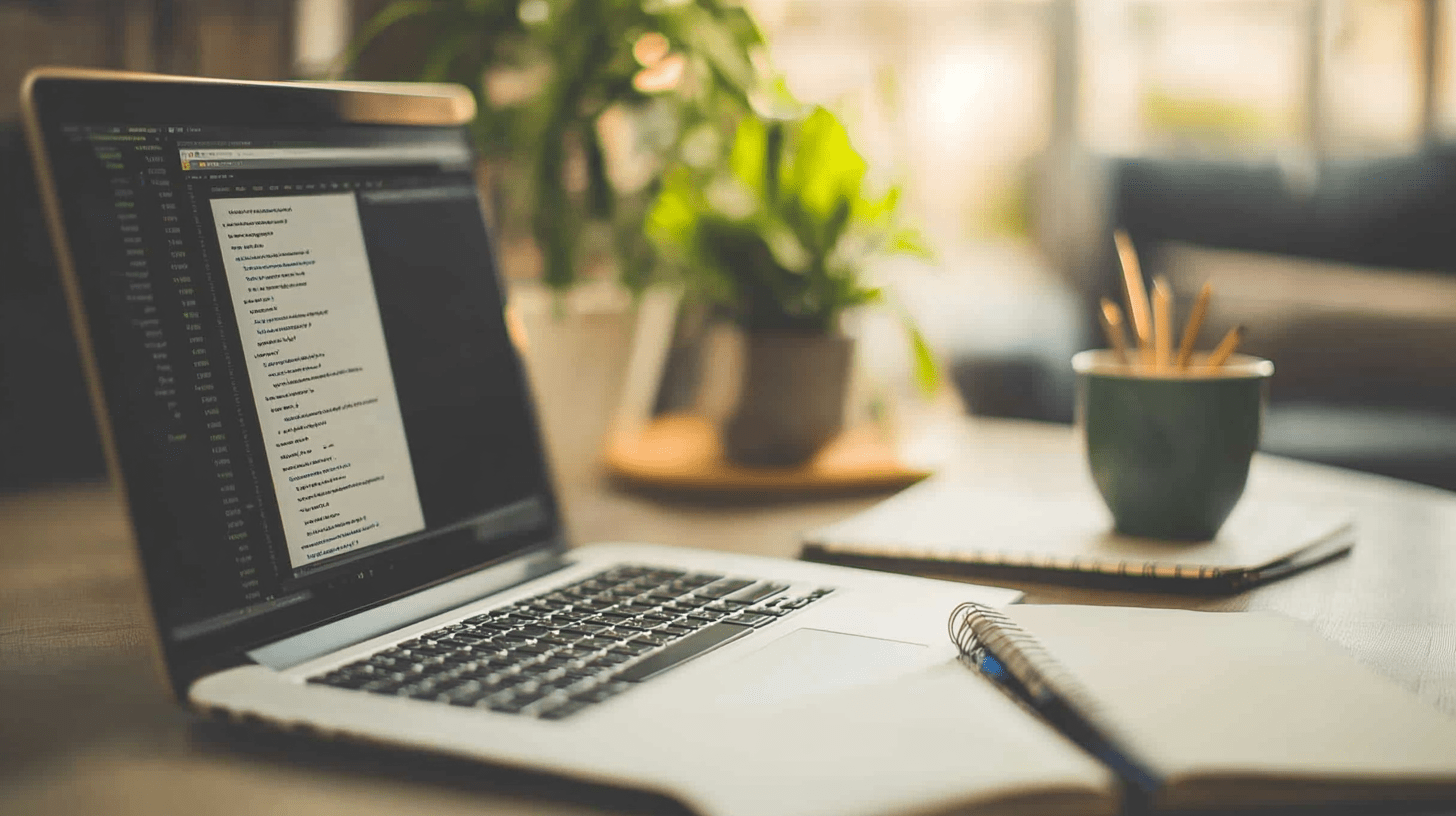
Creating a Custom WordPress Plugin: A Beginner's Guide
This article dives into the world of WordPress plugin development, guiding you through the process from start to finish. Whether you're a business owner looking to customize your site or a developer aiming to expand your skill set, this guide has you covered.
Introduction to WordPress Plugin Development
Creating a custom WordPress plugin can significantly enhance your website's functionality, giving you the freedom to tailor your online presence exactly to your needs. As a seasoned developer and consultant, I've seen firsthand how plugins can transform a standard WordPress site into a powerhouse of custom features. In this guide, my team and I will walk you through the essentials of creating a WordPress plugin, from the basics to more advanced techniques.
Why Create a Custom WordPress Plugin?
Before diving into the how, let's explore the why:
- Customization: Tailor your site to meet specific business needs or user experiences.
- Control: Gain full control over your site's functionality without relying on third-party plugins.
- Performance: A custom plugin can be optimized for your specific use case, reducing bloat and improving site speed.
- Security: Custom plugins can be designed with security in mind, reducing vulnerabilities.
For more on how AI can enhance your WordPress development process, check out our article on /blog/top-ai-tools-for-enhancing-wordpress-development.
The Basics of Plugin Structure
Directory Structure
Here's a basic directory structure for a WordPress plugin:
my-plugin/
├── includes/
│ └── custom-functions.php
├── assets/
│ ├── js/
│ └── css/
├── plugin.php
└── readme.txt
- plugin.php: This is the main file where you define your plugin's header information.
- includes/: For additional PHP files that might be required.
- assets/: For static files like CSS and JavaScript.
Plugin Header
Every WordPress plugin starts with a header in the main PHP file:
<?php
/*
Plugin Name: My Custom Plugin
Description: A brief description of what your plugin does.
Version: 1.0
Author: Ben Bond
Author URI: https://benbond.dev
*/
Writing Your First Function
Let's create a simple function to add a custom shortcode:
function my_custom_shortcode() {
return 'This is my custom shortcode!';
}
add_shortcode('my_shortcode', 'my_custom_shortcode');
This code will allow you to use [my_shortcode]
in your WordPress posts or pages, which will then be replaced with "This is my custom shortcode!".
Advanced Plugin Features
Hooks and Filters
WordPress uses hooks and filters to allow developers to modify core functionality:
- Action Hooks: These let you insert custom code at certain points in WordPress's execution.
- Filter Hooks: These allow you to change the data processed by WordPress.
Here's an example of using an action hook:
function my_admin_notice() {
echo '<div class="notice notice-success is-dismissible"><p>Welcome to your custom plugin!</p></div>';
}
add_action('admin_notices', 'my_admin_notice');
Creating a Custom Post Type
If you need a new type of content, like a "Portfolio" or "Testimonial", you can create custom post types:
function custom_post_type() {
register_post_type('portfolio',
array(
'labels' => array('name' => __('Portfolio')),
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
)
);
}
add_action('init', 'custom_post_type');
For more on integrating custom post types with Next.js for better SEO, see our guide on /blog/creating-custom-post-types-in-wordpress-for-developers.
Best Practices for Plugin Development
- Security: Always sanitize and validate user inputs to prevent SQL injections and XSS attacks.
- Performance: Minimize database queries, use transients for caching, and optimize your code.
- Code Quality: Follow WordPress coding standards, comment your code, and keep it clean.
- Documentation: Write a comprehensive readme.txt file, and document your functions.
Testing and Debugging
Before releasing your plugin:
- Use the WordPress Debug Bar or WP Debugging plugin to catch errors.
- Test on different WordPress versions and themes.
- Ensure compatibility with popular plugins.
Conclusion
Creating a custom WordPress plugin not only allows for unique customization but also positions you as an expert in your field. With the knowledge shared here, you're now equipped to start developing your own plugins. For more advanced topics, consider exploring our articles on /blog/how-to-build-custom-gutenberg-blocks-with-react or /blog/understanding-rest-api-integration-in-wordpress-development.
Remember, if you're looking for professional assistance or want to discuss your plugin ideas, my team and I at Ben Bond are here to help. Feel free to get a quote or contact us for tailored solutions that can propel your online presence forward.
Explore our services for more on how we can help you with your WordPress and Next.js development needs: Ben Bond Services