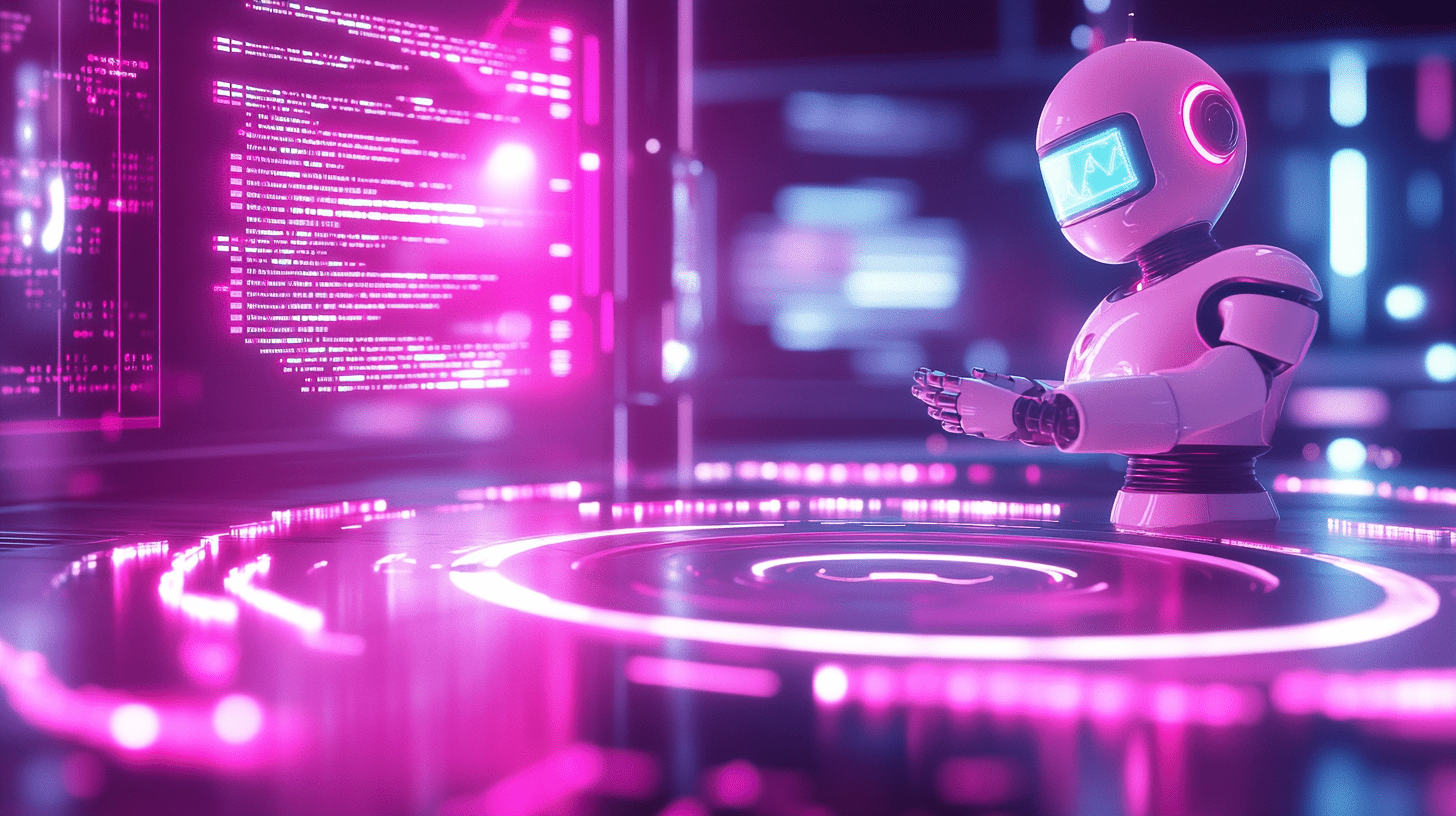
Best Practices for WordPress API Integration with Next.js
Integrating WordPress with Next.js is a powerful strategy for creating fast, SEO-friendly, and dynamic web applications. Here's a comprehensive guide on how to do it effectively, ensuring your site is both performant and scalable.
Why Integrate WordPress with Next.js?
Integrating WordPress with Next.js can revolutionize your web development process. Here are some compelling reasons:
- Speed and Performance: Next.js provides server-side rendering, which speeds up the initial load time, something WordPress can benefit from significantly.
- SEO Advantages: Next.js's static site generation (SSG) and server-side rendering (SSR) capabilities can improve SEO by allowing for faster page loads and better indexing by search engines.
- Scalability: Next.js helps in scaling your application with ease, managing complex routing and API calls more efficiently than traditional WordPress setups.
- Modern Web Development: Utilize React's ecosystem for building interactive UIs, which is particularly useful when creating dynamic content from WordPress.
Best Practices for WordPress API Integration with Next.js
1. Use the WordPress REST API
The WordPress REST API is your gateway to integrating WordPress with Next.js. Here’s how to leverage it:
- Setup: Ensure your WordPress installation has the REST API enabled. Most modern WordPress setups include this by default, but double-check with your host or in your
wp-config.php
. - Authentication: Use OAuth or JWT for secure API calls. Here's a quick setup guide:
**Authentication Example**
- Install the `JWT Authentication for WP-API` plugin.
- Configure the plugin to generate JWT tokens for API requests.
2. Optimize API Requests
Efficient API calls are crucial for performance:
- Batching: Group multiple API requests into one to reduce the number of calls. Here's how:
**Example of Batching in Next.js**
```javascript
const posts = await fetch('https://example.com/wp-json/wp/v2/posts?per_page=100');
const categories = await fetch('https://example.com/wp-json/wp/v2/categories');
- Caching: Implement caching strategies to store API responses locally or use Next.js's built-in caching mechanisms:
**Next.js Caching Example**
```javascript
export async function getStaticProps() {
// Fetch data from WordPress API
const res = await fetch('https://example.com/wp-json/wp/v2/posts');
const posts = await res.json();
return {
props: {
posts,
},
// Revalidate every 10 minutes
revalidate: 600,
}
}
3. Server-Side Rendering (SSR) and Static Site Generation (SSG)
- SSR: Use Next.js’s
getServerSideProps
for pages that need real-time data:
export async function getServerSideProps(context) {
const res = await fetch('https://example.com/wp-json/wp/v2/posts');
const posts = await res.json();
return { props: { posts } };
}
- SSG: For static content, leverage
getStaticProps
to pre-render pages at build time:
export async function getStaticProps() {
const res = await fetch('https://example.com/wp-json/wp/v2/pages/1');
const page = await res.json();
return { props: { page } };
}
4. Handling Dynamic Routes
For dynamic content like blog posts, use Next.js’s dynamic routing:
**Dynamic Routes Example**
```javascript
// pages/posts/[id].js
export async function getStaticPaths() {
const res = await fetch('https://example.com/wp-json/wp/v2/posts');
const posts = await res.json();
const paths = posts.map(post => ({
params: { id: post.id.toString() },
}));
return { paths, fallback: false }
}
export async function getStaticProps({ params }) {
const res = await fetch(`https://example.com/wp-json/wp/v2/posts/${params.id}`);
const post = await res.json();
return { props: { post } };
}
5. Security Considerations
- API Authentication: Ensure all API requests are authenticated, especially when handling sensitive data or user interactions.
- Sanitization: Always sanitize and validate data coming from WordPress to prevent security vulnerabilities.
6. Testing and Debugging
- Local Development: Use tools like
wp-env
or Docker for local WordPress setups to mimic production environments. - Mocking: For development, mock API responses to simulate WordPress data without actual API calls.
7. Deployment Strategies
- Vercel: Deploy your Next.js application with ease on Vercel, which supports both SSG and SSR.
- CI/CD: Set up continuous integration and deployment pipelines to automate the build and deployment process.
Conclusion
Integrating WordPress with Next.js isn't just about combining two technologies; it's about leveraging the strengths of both to create a robust, fast, and SEO-friendly web application. By following these best practices, you can ensure your project benefits from the content management prowess of WordPress and the performance capabilities of Next.js.
My team and I at Ben Bond have extensive experience in this area, and we're here to help you navigate these integrations. Whether you need advice, hands-on development, or optimization services, feel free to contact us or request a quote to see how we can assist you in building your next project.
For more insights on WordPress and Next.js development, explore our other articles like:
- How to Build Scalable WordPress Sites with Next.js
- Creating a Custom WordPress Plugin: A Beginner's Guide
Remember, with the right approach, integrating WordPress with Next.js can significantly enhance your web development capabilities, providing a seamless experience for both developers and users alike.