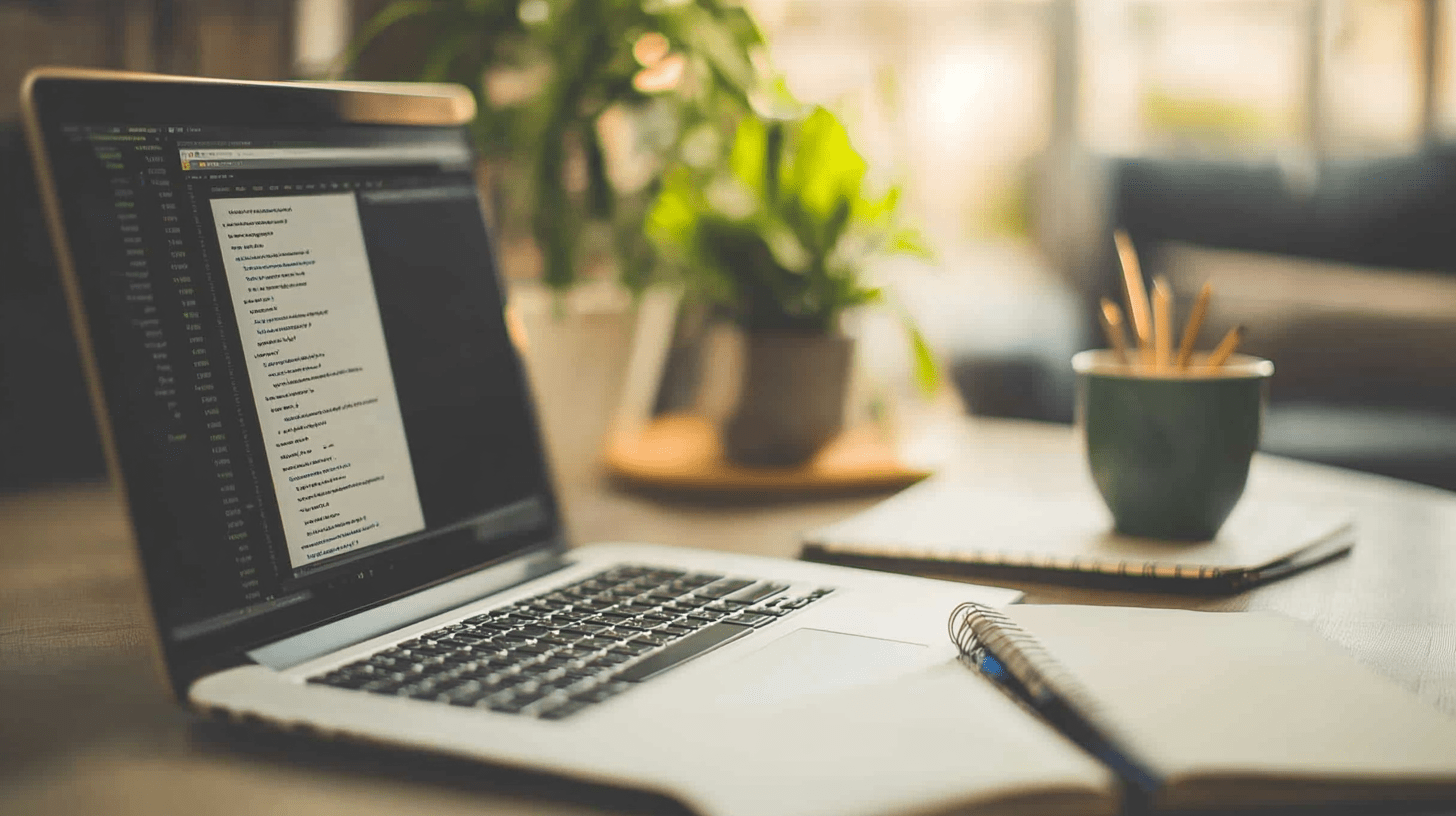
Creating Dynamic Web Pages in Next.js for Better SEO
Unlock the Power of Dynamic Web Pages with Next.js for Enhanced SEO
In the ever-evolving world of web development, staying ahead in search engine rankings is crucial for any business or developer. Here at Ben Bond's consultancy, my team and I have mastered the art of creating dynamic web pages using Next.js, which not only improves user experience but also significantly enhances SEO. Let's dive into how you can leverage Next.js to make your web pages not just dynamic but also SEO-friendly.
Why Choose Next.js for Dynamic Web Pages?
Next.js, developed by Vercel, is a React framework that enables you to create server-side rendered (SSR) and statically generated (SSG) applications with ease. Here are the key reasons why my team and I recommend Next.js:
SEO-Friendly by Design: Next.js allows for server-side rendering, which means your pages can be indexed by search engines even before users visit them. This is crucial for SEO as it ensures content is crawlable and indexable.
Dynamic Routes: With Next.js, you can create dynamic routes that load content from an API or database, offering a seamless user experience and making your site more interactive.
Fast Load Times: Next.js optimizes load times through techniques like code splitting, automatic image optimization, and more, which are essential for SEO as Google uses page speed as a ranking factor.
Better User Experience: Dynamic content loading and optimized performance lead to a better user experience, which indirectly affects SEO through increased engagement and lower bounce rates.
How to Create Dynamic Web Pages in Next.js
Let's walk through the steps to create dynamic web pages that are SEO-optimized:
1. Setup Your Next.js Project
Start by setting up a new Next.js project:
npx create-next-app@latest my-next-app
cd my-next-app
2. Create Dynamic Routes
Dynamic routes in Next.js are created by adding brackets around the dynamic part of the filename. For example:
pages/posts/[id].js
This file will handle all URLs like /posts/1
, /posts/2
, etc. Here's a simple example:
import { useRouter } from 'next/router'
function Post() {
const router = useRouter()
const { id } = router.query
return <p>Post: {id}</p>
}
export default Post
3. Fetch Dynamic Content
To fetch dynamic content:
export async function getServerSideProps(context) {
const res = await fetch(`https://api.example.com/posts/${context.params.id}`)
const post = await res.json()
return {
props: {
post,
},
}
}
This function will run at request time, ensuring that the content is always up-to-date, which is perfect for SEO.
4. Optimize for SEO
- Use
<Head>
for Metadata: Insert SEO metadata like title, description, and keywords:
import Head from 'next/head'
function Post({ post }) {
return (
<div>
<Head>
<title>{post.title}</title>
<meta name="description" content={post.excerpt} />
</Head>
<h1>{post.title}</h1>
<div>{post.content}</div>
</div>
)
}
- Schema Markup: Implement schema markup to help search engines understand the context of your content:
import { NextSeo } from 'next-seo'
function Post({ post }) {
return (
<div>
<NextSeo
title={post.title}
description={post.excerpt}
openGraph={{
title: post.title,
description: post.excerpt,
}}
/>
{/* Rest of the component */}
</div>
)
}
5. Leverage Next.js Features for SEO
- Automatic Image Optimization: Use Next.js's built-in image optimization to reduce load times:
import Image from 'next/image'
function Post({ post }) {
return (
<div>
<Image
src={post.image}
alt={post.title}
width={500}
height={300}
layout="responsive"
/>
</div>
)
}
Server-Side Rendering (SSR) or Static Site Generation (SSG): Choose based on your content update frequency:
- SSR for content that changes frequently.
- SSG for static content, which can be pre-rendered at build time for better SEO.
SEO Best Practices with Next.js
Here are some additional SEO tips:
- Use Canonical URLs to avoid duplicate content issues.
- Implement Proper Redirects for URL changes to maintain SEO value.
- Optimize for Mobile as Google uses mobile-first indexing.
- Regularly Update Content to keep your site relevant and engaging.
Conclusion
Creating dynamic web pages with Next.js not only enhances user experience but also significantly boosts your site's SEO. By following these steps and leveraging Next.js's powerful features, my team and I at Ben Bond's consultancy can help you craft a site that not only looks great but also ranks well in search engines.
If you're interested in taking your web development to the next level, check out our services at benbond.dev/services, get a personalized quote at benbond.dev/quote, or reach out to us directly at benbond.dev/contact.
Remember, with Next.js, you're not just building a website; you're crafting an SEO powerhouse. Let's make your site dynamic, user-friendly, and highly visible in search results together.
For more insights on how to integrate AI into your Next.js projects, explore our article on AI-powered SEO strategies for Next.js sites. If you're curious about how AI can revolutionize WordPress development, dive into How AI is transforming web development in 2024. And for a deep dive into Next.js and WordPress integration, don't miss A step-by-step guide to building a headless WordPress site with Next.js.